If you use the Salesforce APIs to solve non-trivial problems, you will eventually get an error which looks like:
[UnexpectedErrorFault [ApiFault exceptionCode=’UNKNOWN_EXCEPTION’
exceptionMessage=’An unexpected error occurred. Please include this ErrorId if you contact support: 844179212-45324 (-195209423)’
extendedErrorDetails='{[0]}’
What do you think happens if you contact support and provide them with the magic ErrorId 844179212-45324 (-195209423)?
- Salesforce support thanks you profusely, sends you a Salesforce polo, and makes solving your issue a top priority.
- Salesforce support asks you what 844179212-45324 (-195209423) means.
- Salesforce support asks for more information.
- Salesforce support tells you what the issue may be based on the ErrorId.
- Salesforce support tells you that there is probably a bug in your code.
This article is about what you can do to if the answer you get is anything but answer #1 (If you have figured out how to consistently get #1 as the answer I would like to know your secret!)
We Have Seen a Lot of UNKNOWN_EXCEPTIONs
In my day job, we see an UNKNOWN_EXCEPTION at least once or twice every month and often the “unknown” follows no perceivable logical pattern.
- An UNKNOWN_EXCEPTION may crop up on a single ECMA instance but not anywhere else in the world.
- An UNKNOWN_EXCEPTION may occur on a standard Salesforce table on some Salesforce instances but not all.
- An UNKNOWN_EXCEPTION may occur today but mysteriously go away a few days later.
- An UNKNOWN_EXCEPTION may occur when querying a table BUT only for certain records.
- An UNKNOWN_EXCEPTION may occur when inserting into a standard table, but not all of the time.
Unless you are brave (or forced into bravery) or want to read this entire post, the easiest thing to do may be:
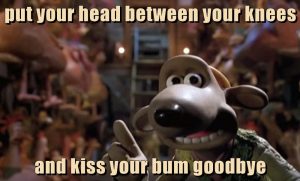
There is hope! If you will spend time to isolate an UNKNOWN_EXCEPTION to a small bit of SOQL (or something else small), our experience is that Salesforce support will quickly act and solve the issue (usually within a few days).
UNKNOWN Cause #1: SOQL SELECT UNKNOWN_EXCEPTIONs
The #1 cause of UNKNOWN_EXCEPTIONS is with SOQL SELECT statements, at least in my 10+ years of bumping into the “unknown.” Of the issues we have seen and solved, they have fallen into one of the following categories.
- Selecting a specific column throws an UNKNOWN_EXCEPTION.
- Selecting records in a specific time range and a specific column throws an UNKNOWN_EXCEPTION.
- Selecting a base64 column from a specific record using SOAP throws an UNKNOWN _EXCEPTION.
- Selecting a base64 column from a specific record using REST return a 404 or 500 code when fetching the base64 data (the same record returns an UNKNOWN_EXCEPTION when using SOAP).
UNKNOWN Cause #2: SELECT a Specific Column
The SOQL queries we tend to write look like:
- SELECT Id, Name,…. WHERE SystemModStamp >= startOfRange and SystemModStamp <= endOfRange LIMIT 20000
The steps to isolate an UNKNOWN_EXCEPTION are below. This will generate something manageable for Salesforce support.
- Start removing columns from the query until only the problem column remains.
- Start shrinking the timestamp interval until it is down to a few seconds (or milliseconds).
- Run the query in the Salesforce developer console and watch the exception happen.
- Submit a support ticket to Salesforce.
In practice the problem column has been a formula or a custom field nearly 100% of the time. Trying to the guess the problem column is a waste of time — be methodical and cut the columns in half and do a binary search for the problem.
What is the root cause? Technically, of course, we cannot know for sure but our experience says a corrupted index or data block in Oracle is to blame.
If you hit this issue more than a few times, then you will probably what do to what we did — write code which does the analysis and computes minimal SOQL needed to exhibit the UNKNOWN_EXCEPTION. It can take quite a few SOQL queries to find the problem for wide tables — computers are more patient is doing this sort of work than me!
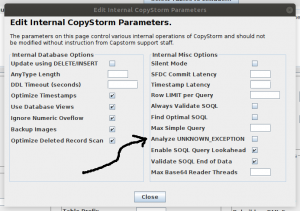
UNKNOWN Cause #3: SELECT a Specific Time Range
The SOQL queries we tend to write look like:
- SELECT Id, Name,…. WHERE SystemModStamp >= startOfRange and SystemModStamp <= endOfRange LIMIT 20000
and sometimes an UNKNOWN_EXCEPTION occurs ONLY within a specific range of records. MOST OF THE TIME this problem also includes a problem column but not always. When you try:
- Start removing columns from the query until only the problem column remains.
and the problem does not go away even when selecting Id or any other single column (or possibly just Id) then you are facing a SQOL SELECT Timestamp range issue.
Salesforce support will want to see a simple SOQL query with a tiny timestamp range. Use the developer console to reduce your problem query to something like:
- SELECT Id, AnyColumn FROM Account WHERE SystemModStamp >= 2018-01-01T00:00:00.000Z AND SystemModStamp <=2018-01-01T00:00:01.000Z
and submit the issue to Salesforce support.
What is the root cause? Technically, of course, we cannot know for sure but our experience says a corrupted index or data block in Oracle.
UNKNOWN Cause #4: Disappearing UNKNOWN_EXCEPTION
Surprisingly, an UNKNOWN_EXCEPTION sometimes appears and then goes away in a few days. In our experience this occurs 10-20% of the time and it does not matter whether a support issue is filed with Salesforce. Kudos to Salesforce for looking at their error logs and fixing problems.
If your situation is not time critical then this may your first line of response. It is easy, does not take any of your time, and sometimes works.
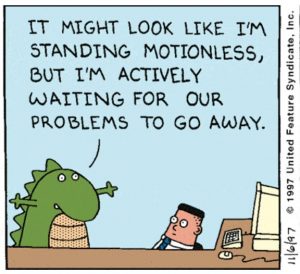
UNKNOWN Cause #5: BASE64 Related UNKNOWN_EXCEPTION
Getting an UNKNOWN_EXCEPTION when reading a BASE64 column seems to be a rare case but one we have seen multiple times with customers using Shield. This is a bad one because it tends to indicate that data is missing from Salesforce’s content storage subsystem.
If you see this sort of error, there are a few extra steps:
- Use the REST API to read the record. The REST API will provide a hyperlink to the data stored in Salesforce’s content storage subsystem.
- Try the hyperlink using CURL — if there is a problem the request will fail with a 404 or 500 response code from Salesforce.
- Try looking for the document in the Salesforce GUI. It should fail.
- File a issue with Salesforce support.
If you hit this error, it is wise to systematically search your Salesforce instance for other records with the same problem. You can write your own code or use a free tool, CopyStormSanityChecker, to check all base64 columns. Send support@capstorm.com an email for the newest download links.